In a folder, you should be able to rely on the extension of the file. For example, only pick up xml files: c:\some\path\*.xml
Or only pick up JSON files: c:\some\path\*.json
In sometime cases you don't have an extension. So, you would need to set your Source message type to Text (ISO-8859-1 encoding) or Binary, and then determine the type programmatically.
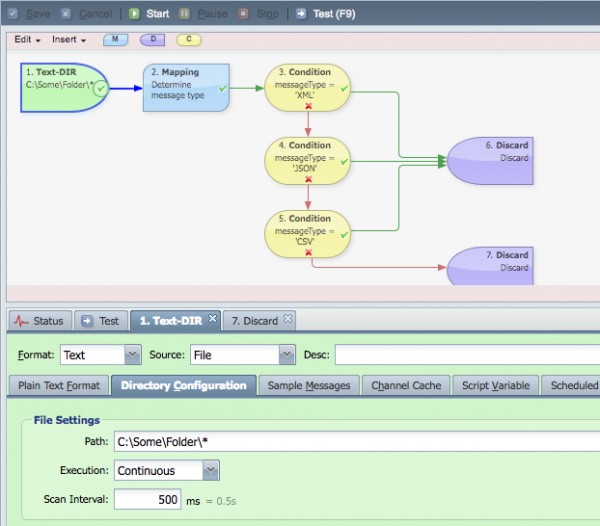
Then in a mapping node, you can attempt to parse the message into the different types. Here is the script you could use:
var messageType = "UNKNOWN";
var newMessage;
try {
newMessage = qie.parseXMLString(source.getNode("/"));
message = qie.createXMLMessage(source.getNode("/"), 'UTF-8');
messageType = "XML";
} catch (xmlErr) {
qie.debug("Caught this XML error: " + xmlErr);
try {
newMessage = qie.parseJSONString(source.getNode("/"));
message = qie.createJSONMessage(source.getNode("/"), 'UTF-8');
messageType = "JSON";
} catch (jsonErr) {
qie.debug("Caught this JSON error: " + jsonErr);
try {
newMessage = qie.parseHL7String(source.getNode("/"));
message = qie.createHL7Message();
message.setNode("/", source.getNode("/"));
messageType = "HL7";
} catch (hl7Err) {
qie.debug("Caught this HL7 error: " + hl7Err);
try {
newMessage = qie.parseCSVString(source.getNode("/"));
message = qie.createCSVMessage();
message.setNode("/", source.getNode("/"));
messageType = "CSV";
} catch (csvErr) {
qie.debug("Caught this CSV error: " + csvErr);
messageType = "UNKNOWN";
}
}
}
}
qie.debug("Determined Message Type is " + messageType);
messageCache.setValue('messageType', messageType);
Here is the exported config for my test channel:
https://www.qvera.com/kb/?qa=blob&qa_blobid=9553839279022932067