Assuming that you are wanting to calculate a modulus-256 checksum, you could add the following published functions to your configuration. Then you just pass the string that you want the checksum calculated on into the fucntion and the output will be an uppercase hexidecimal checksum.
function calcASTMChecksum(inputValue) {
// calculate the modulo 256 checksum
var sum = 0;
var len = inputValue.length;
for (var i = 0; i < len; i++) {
sum += inputValue.charCodeAt(i);
if (sum & 0x80) {
sum = (0xff - sum + 1)*-1;
}
}
sum = sum % 256;
if (sum < 0) {
sum *= -1;
}
// convert to a two byte uppercase hex value
var chars = sum.toString(16).toUpperCase();
if (chars.length == 1) {
chars = "0" + chars;
}
return chars;
}
If you are receiving the messages using a socket, you will configure the source to respond "From Mapping or Destination node".
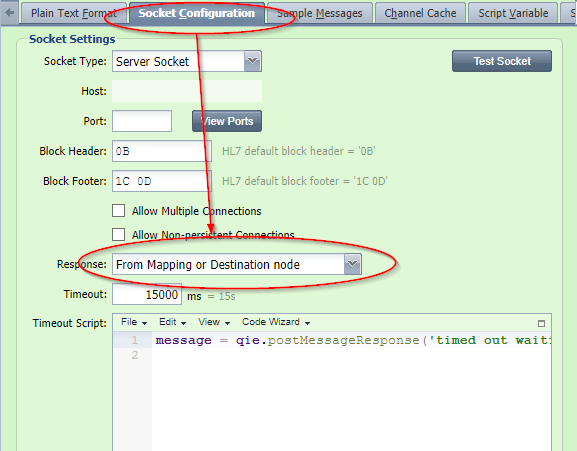
Then from a mapping script you can build the bytes for the response and respond using the qie.postMessageResponse() method.